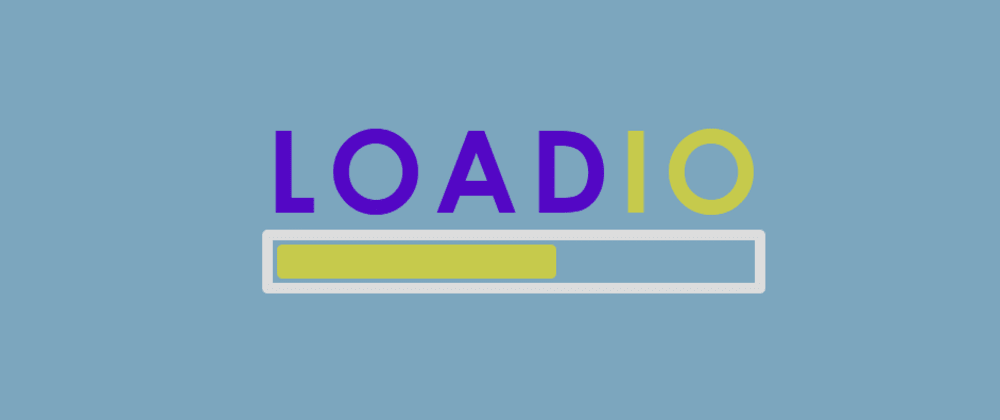
Introduction
Many projects contain asynchronous calls. The operation of these may be unaware of the user, or the user may need to know about that status.
In order to notify the user of this, the loading component is shown on the screen and the user is informed that something is running. At this point, the management of asynchronous methods should be managed in various ways and the component should be demonstrated.
Today, I will show you how you can handle this in an easy way with loadio.
loadio
This package is a simple to use tool that allows you to manage status information with promises.
Install it with:
import { withPool, useStatus } from "loadio"; const fetch = withPool(global.fetch);
Wrap the method you want to follow the status information.
import { withPool, useStatus } from "loadio"; const fetch = withPool(global.fetch);
Or add promise directly into it with PoolManager
PoolManager.append(fetch("get/data"));
And that’s all. You can easily view the status on your home page by calling the new method you have wrapped in place of the old one.
import { useStatus } from "loadio";
function HomePage({ isLoading }) {
const status = useStatus();
return (
<>
{isLoading ? "Loading..." : "Loaded!"}
<button onClick={() => myWrappedfetch("get/data")}>Get</button>
</>
);
}
It also generates a percentage of information according to the number of tasks.
{
isLoading?: boolean,
percentage?: number,
runningTasks?: number
}
A complete example with React Component is as follows.
import React from "react";
import { withPool, useStatus } from "loadio";
const fetch = withPool(global.fetch);
class HomePage extends React.Component {
render(){
const { isLoading, percentage } = this.props;
return (
<>
{isLoading ? "Loading..." : "Loaded!"}
{percentage + "%"}
<button onClick={() => fetch("get/data")}>Get</button>
</>
);
}
}
export default withStatus(HomePage);
Demo
@Credit Dev.to